
하드웨어
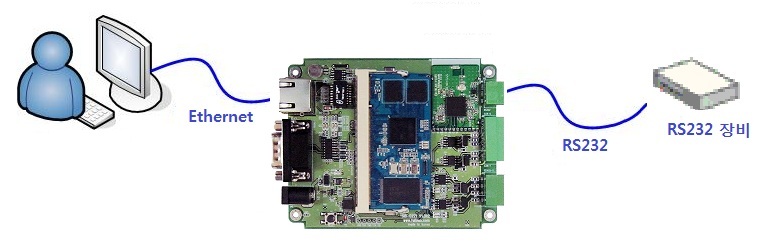
#include <stdio.h> #include <arpa/inet.h> #include <termios.h> // B115200, CS8 등 상수 정의
#define BUFF_SIZE 1024
int fd_server; // TCP 서버 소켙의 파일 디스크립터 struct termios newtio;
void on_data() rd_cnt = read ( fd_client, buff_rcv, BUFF_SIZE); // 클라이언트로부터 전송된 메시지 수령
void on_accept()
// 여기까지 실행되었다면 클라이언트로부터 접속 요청이 있었음 if ( -1 == fd_client ) {
if ( 0 == poll_events[1].fd ) {
int main( int argc, char *argv[])
// RS232 포트 open //////////////////////////////////////////////////////////// fd_232 = open( argv[1], O_RDWR | O_NOCTTY | O_NONBLOCK ); // 디바이스를 open 한다.
memset( &newtio, 0, sizeof(newtio) ); tcflush (fd_232, TCIFLUSH );
// TCP 서버 //////////////////////////////////////////////////////////////// struct sockaddr_in addr_in; fd_server = socket( PF_INET, SOCK_STREAM, 0 );
option = 1; bzero( &addr_in, sizeof( struct sockaddr_in ) ); if( bind( fd_server, (struct sockaddr *)&addr_in, sizeof( struct sockaddr_in ) ) < 0 ) {
if ( 0 > listen( fd_server, 1 ) ) {
// poll 초기화 및 등록 //////////////////////////////////////////////////// poll_events[0].fd = fd_server; // 서버 소켙의 파일 디스크립터 저정
// 자료 송수신 ////////////////////////////////////////////////////////////
poll_state = poll( // poll()을 호출하여 event 발생 여부 확인
if ( 0 < poll_state ) { // 발생한 event 가 있음 if ( 0 == ndx_poll ) { if ( poll_events[ndx_poll].revents & POLLERR ) { // event 가 에러? close( fd_server); |
다음 강좌에서는 Ethernet의 UDP로 들어온 데이타를 RS232로 보내는 프로그램을 설명하도록 하겠습니다.