
하드웨어
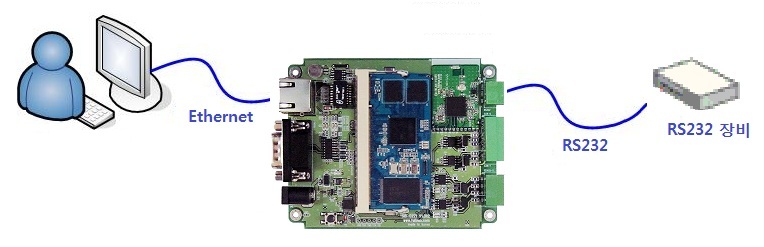
#include <stdio.h> #include <stdlib.h> #include <string.h> #include <unistd.h> #include <sys/types.h> #include <sys/poll.h> #include <termios.h> // B115200, CS8 등 상수 정의 #include <fcntl.h> // O_RDWR , O_NOCTTY 등의 상수 정의 #include <sys/socket.h> #include <arpa/inet.h> int main( int argc, char *argv[]) { int fd; int ndx; int cnt; char buf[1024]; struct termios newtio; struct pollfd poll_events; // 체크할 event 정보를 갖는 struct int poll_state; int tcp_client_socket; int server_addr_size; struct sockaddr_in tcp_server_addr;
if ( 3 > argc){ printf( "인수가 모자릅니다. 시리얼 포트를 입력하여 주시오\n"); printf( "실행방법: ]$ ./app_rs232_to_tcp /dev/ttySAC2 127.0.0.1\n"); exit( 0); } // 시리얼 포트를 open fd = open( argv[1], O_RDWR | O_NOCTTY | O_NONBLOCK ); // 디바이스를 open 한다. if ( 0 > fd){ printf("open error\n"); return -1; } // 시리얼 포트 통신 환경 설정 memset( &newtio, 0, sizeof(newtio) ); newtio.c_cflag = B115200 | CS8 | CLOCAL | CREAD; newtio.c_oflag = 0; newtio.c_lflag = 0; newtio.c_cc[VTIME] = 0; newtio.c_cc[VMIN] = 1; tcflush (fd, TCIFLUSH ); tcsetattr(fd, TCSANOW, &newtio ); fcntl(fd, F_SETFL, FNDELAY); // poll 사용을 위한 준비 poll_events.fd = fd; poll_events.events = POLLIN | POLLERR; // 수신된 자료가 있는지, 에러가 있는지 poll_events.revents = 0; // tcp 소켓 연결 tcp_client_socket = socket( PF_INET, SOCK_STREAM, 0); if( -1 == tcp_client_socket){ printf( "socket 생성 실패n"); exit( 1); } memset( &tcp_server_addr, 0, sizeof( tcp_server_addr)); tcp_server_addr.sin_family = AF_INET; tcp_server_addr.sin_port = htons( 4000); tcp_server_addr.sin_addr.s_addr = inet_addr( argv[2]);
// 자료 송수신 while ( 1) { poll_state = poll( // poll()을 호출하여 event 발생 여부 확인 (struct pollfd*)&poll_events, // event 등록 변수 1, // 체크할 pollfd 개수 1000 // time out 시간 ); if ( 0 < poll_state) { // 발생한 event 가 있음 if ( poll_events.revents & POLLIN) { // event 가 자료 수신? cnt = read( fd, buf, 1024); buf[cnt] = '\0'; printf( "data received - %d %s\n", cnt, buf); sendto( tcp_client_socket, buf, strlen( buf)+1, 0, // +1: NULL까지 포함해서 전송 ( struct sockaddr*)&tcp_server_addr, sizeof( tcp_server_addr)); close( tcp_client_socket); }
if ( poll_events.revents & POLLERR) { // event 가 에러? printf( "통신 라인에 에러가 발생, 프로그램 종료"); break; } } }
close( fd); return 0;
}
|
다음 강좌에서는 C221-S3C6410 보드에 있는 Eternet과 RS232 을 이용하여
Ethernet의 TCP로 들어온 데이타를 RS232로 보내는 프로그램을 설명하도록 하겠습니다.